* Concurrenty (동시성)
- 사용자 인터랙션 처리 (Main Thread에서 처리)
- 네트워킹
- 백그라운드에서 파일 다운로드
- 파일 저장하고 읽기
컴퓨터에서는 여러 개의 스레드가 동시에 수행되면서 백그라운드에서 수많은 작업들이 이루어진다.
* GCD (Grand Centeral Dispatch)
: 해야 할 일들을 GCD로 전달해주면 시스템에서 안전하게 수행될 수 있도록 처리 (Queue FIFO 구조로 처리)
* DispatchQueue
1) Main Queue : 메인 스레드에서 작동하는 큐
ex) DispatchQueue.main.async { ... }
2) Global Queue : 시스템에 의해 관리되는 큐
# QoS (Quality of Service) : 큐의 우선순위를 결정 지음
# 우선순위 중요도
userInteractive > userInitiated > dafault > utility > background
ex) Dispatch.global(qos: .background).async { ... }
3) Custom Queue
ex) let concurrentQueue = DispatchQueue(label: "concurrent", sos: .background, attributes: .concurrent)
4) 두 개의 Queue을 같이 쓰기 (실무에서 자주 사용할 것으로 예상)
ex) 이미지 파일 다운로드 이후, 보여주기
* Sync (동기) & Async (비동기)
- sync : 동기 작업이 완료되기 전까지 다음 작업은 Block(멈춤) 상태
- async : 현재 수행 중인 작업이 동작 중이라도, 다음 작업이 중간에 끼어들 수 있는 상태
# 실제 앱에서는 async를 많이 사용한다. 다만, 선행~후행 작업이 인과관계가 있다면 상황에 따라 sync를 사용하기도 한다.
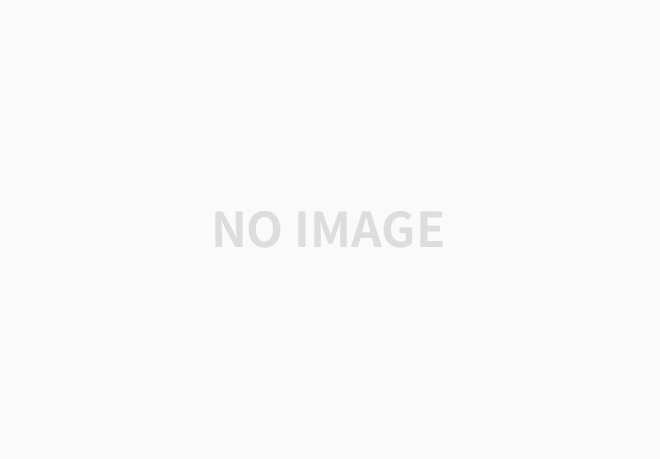
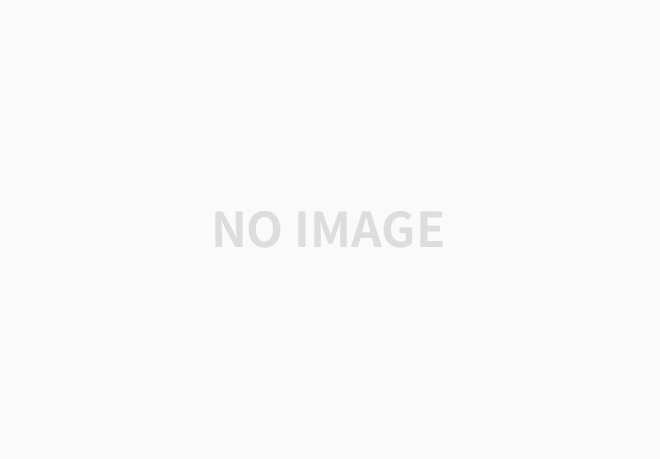
< 예제 소스 >
import UIKit
// Queue - Main, Global, Custom
// - Main Queue : UI나 사용자 인터렉션 핸들러 관련해서 사용
DispatchQueue.main.async {
// UI update
let view = UIView()
view.backgroundColor = #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0)
}
// - Global Queue :
DispatchQueue.global(qos: .userInteractive).async {
// 1순위 (바로 시작해야 함)
}
DispatchQueue.global(qos: .userInitiated).async {
// 2순위 (사용자가 결과를 기다림)
}
DispatchQueue.global(qos: .default).async {
// 기본값이므로 굳이 선언할 필요는 없음
// DispatchQueue.global() 일반함수 형태와 동일
}
DispatchQueue.global(qos: .utility).async {
// 사용자가 급하지 않은 업무를 등록 (Ex. 큰 파일 다운로드, 네트워킹)
}
DispatchQueue.global(qos: .background).async {
// 사용자에게 당장 인식될 필요가 없는 것들 (Ex. GPS 위치 업데이트, 날씨/뉴스데이터 미리 받기)
}
// - Custom Queue : 직접적으로 관리해줘야 하는 경우
let concurrentQueue = DispatchQueue(label: "concurrent", qos: .background, attributes: .concurrent)
let serialQueue = DispatchQueue(label: "serial", qos: .background)
// 복합적인 상황 (Ex. 이미지 파일을 다운로드 받고, 이후에 이미지를 보여줌)
func donwloadImageFromServer() -> UIImage {
return UIImage()
}
func updateUI(image: UIImage) {
}
DispatchQueue.global(qos: .background).async {
// download
let image = donwloadImageFromServer()
DispatchQueue.main.async {
// update UI
updateUI(image: image)
}
}
// Sync, Async
// Async
//DispatchQueue.global(qos: .background).async {
// for i in 0...5 {
// print ("😈 \(i)")
// }
//}
//
//DispatchQueue.global(qos: .userInteractive).async {
// for i in 0...5 {
// print ("😙 \(i)")
// }
//}
DispatchQueue.global(qos: .background).sync {
for i in 0...5 {
print ("😈 \(i)")
}
}
DispatchQueue.global(qos: .userInteractive).async {
for i in 0...5 {
print ("😙 \(i)")
}
}
'iOS' 카테고리의 다른 글
[27일차] Codable 이란? (0) | 2020.08.19 |
---|---|
[26일차] URLSession (꼭 알아야 할 개념) (0) | 2020.08.16 |
[24일차] Network 통신 (0) | 2020.08.14 |
[23일차] TodoList 추가/삭제 (0) | 2020.08.12 |
[22일차] InputText 와 소프트 키보드 영역 (0) | 2020.08.11 |
댓글